この記事の内容
この記事では、Pythonを使用して、テキストファイルの書き込みを行う方法を紹介しています。
基本的な内容で、よく使う処理になりますので、確実におさえておく必要のある項目です。
この記事を読むことで、テキストファイルの書き込み処理を学ぶことができます。
テキストファイルの読み込みについては、以下の記事で解説していますので、そちらを参考にしてください。
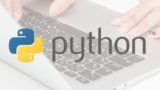
テキストファイルに書き込む方法
書き込みモードでファイルを開く
まずは、書き込みモードでファイルを開き、閉じてみます。
以下の内容のsample.txtを使用します。
This is the second line.
This is the last line.
def main(file_path): with open(file_path, "w") as file: print("ファイルを書き込みモードで開きました") # >> ファイルを書き込みモードで開きました if __name__ == "__main__": file_path = 'sample.txt' main(file_path)
ここでの注意は、“w”で開いた場合、ファイルの内容が消えますので注意してください。
そのため、このプログラム実行後は、上記のsample.txtの内容が消えた状態で書き込まれます。
テキストファイルに文字列を書き込む
次に、指定した文字列をファイルへ書き込みを行います。
def main(file_path): with open(file_path, "w") as file: file.write("This is the first line.") if __name__ == "__main__": file_path = 'sample.txt' main(file_path)
このプログラム実行後に、sample.txtには以下の文字列が書き込まれます。
複数行の文字列をファイルに書き込む
複数行のテキスト書き込みを行います。
複数行書き込む場合は、改行コード(\n)を行末に指定してください。
def main(file_path): with open(file_path, "w") as file: file.write("This is the first line.\n") file.write("This is the second line.\n") if __name__ == "__main__": file_path = 'sample.txt' main(file_path)
このプログラム実行後、sample.txtは以下の内容になります。
This is the second line.
listを元に複数行のテキストを書き込む
listに書き込む文字列を入れ、writelines()関数を用いることで、複数行書き込みができます。
def main(file_path): with open(file_path, "w") as file: content = ["This is the first line.\n", "This is the second line.\n"] file.writelines(content) if __name__ == "__main__": file_path = 'sample.txt' main(file_path)
読み込んだテキストファイルの内容を、別ファイルに書き込む
これまでの応用で、ファイルを読み込むと同時に別ファイルに書き込む方法を紹介します。
以下の内容のsample.txtを使用します。
This is the second line.
This is the last line.
def main(input_file_path, output_file_path): with open(input_file_path, "r") as input_file, \ open(output_file_path, "w") as output_file: lines = input_file.readlines() for line in lines: output_file.write(line) if __name__ == "__main__": input_file_path = 'sample.txt' output_file_path = 'sample_output.txt' main(input_file_path, output_file_path)
このプログラム終了後、sample.txtと同じ以下の内容のsample_output.txtが生成されます。
This is the second line.
This is the last line.
テキストファイルへの追記
テキストファイルへの追記方法について記載します。
ポイントは、open()関数の引数に”a”を指定する部分になります。
これで追記モードで開くことができます。
以下の内容のsample.txtを使用します。
This is the second line.
def main(file_path): with open(file_path, "a") as file: file.write("This is the last line.\n") if __name__ == "__main__": file_path = 'sample.txt' main(file_path)
このプログラム実行後、sample.txtは以下の内容になります。
This is the second line.
This is the last line.
まとめ
この記事では、以下のテキストファイルの書込方法について学びました。
- 書き込みモードでファイルを開く
- テキストを書き込む
- 複数行のテキストを書き込む
- listを元に複数行のテキストを書き込む
- 読み込んだテキストファイルの内容を、別ファイルに書き込む
- テキストファイルへの追記
テキストファイルの扱いは、使用頻度の高い処理になりますので、是非おさえておきましょう。
コメント